Event Bubbling in ASP.NET 2.0 and templates
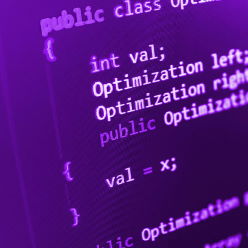
But there is a way to bubble events to the upper controls and catch them. The first thing you should look at is the OnBubbleEvent protected method of the Control object. It catches all the events raised by its controls. Override it and you can catch events and treat them. Now, this method returns a bool value. It tells the RaiseBubbleEvent method (I'll talk about it shortly) if the event was handled or not. If it was, then the event doesn't rise above that control.
Now, about the RaiseBubbleEvent method, also a protected method of the Control object. What it does is go up the object hierarchy and execute on each object the OnBubbleEvent. It also stops if the method returns true. It is so simple and perfect that it is not overridden in any of the ASP.NET 2.0 WebControls.
But there is a catch. Some objects, like DataLists, DataGrids, Gridviews, Repeaters, etc, choose to handle the events in their items no matter what. That's why if you click a button inside a DataList, the event won't bubble to the OnBubbleEvent method from the UserControl or Page the DataList is in. Instead, the event reaches the OnItemCommand event.
So, if you want to use this general bubbling method with WebControls that override OnBubbleEvent, you have two options.
One is use inherited objects that remove this issue altogether (like inheriting from a DataList, overriding OnBubbleEvent, executing base.OnBubbleEvent, then always return false), which might make things cumbersome since you would have to define a control library.
The second option is to always handle the OnItemCommand and other similar events with something like RaiseBubbleEvent(sender, args); . This very line will re bubble the event upwards.
Benefits: the benefits of using this general event bubbling technique is that you don't have to always specifically write code in the OnItemCommand or having to handle OnClick events and so on and so on. Just catch all events, check if their arguments are CommandEventArgs, then handle them depending on source, command name and command argument.