Visual Studio Orcas for Vista RC1 Available
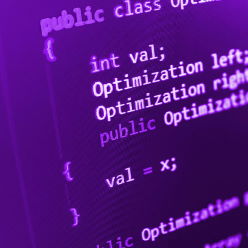
A quick intro interview with Sam Guckenheimer, Lead Product Planner for Orcas:
Orcas and the Future of Visual Studio
protected override AutoGeneratedField CreateAutoGeneratedColumn(
AutoGeneratedFieldProperties fieldProperties)
{
AutoGeneratedField field =
base.CreateAutoGeneratedColumn(fieldProperties);
StateBag sb = (StateBag)field.GetType()
.InvokeMember("ViewState",
BindingFlags.GetProperty |
BindingFlags.NonPublic |
BindingFlags.Instance,
null, field, new object[] {});
sb["DataFormatString"] = "{0:N}"; //or the format string you prefer
field.HtmlEncode=false; //see update
return field;
}
public virtual void VerifyRenderingInServerForm(Control control)
{
if (this.Context == null || base.DesignMode) return;
if (control == null)
{
throw new ArgumentNullException("control");
}
if (!this._inOnFormRender && !this.IsCallback)
{
throw new HttpException(System.Web.SR.GetString("ControlRenderedOutsideServerForm", new object[] {
control.ClientID,
control.GetType().Name
}));
}
}
int customerIDIndex = table.Columns.IndexOf("customerID");
int customerFirstNameIndex = table.Columns.IndexOf("firstName");
int customerLastNameIndex = table.Columns.IndexOf("lastName");
System.Diagnostics.Debug.Assert(customerIDIndex > -1,
"Database out of sync");
System.Diagnostics.Debug.Assert(customerFirstNameIndex > -1,
"Database out of sync");
System.Diagnostics.Debug.Assert(customerLastNameIndex > -1,
"Database out of sync");
foreach(DataRow row in table.Rows){
customer = new Customer();
customer.ID = (Int32)row[customerIDIndex];
customer.FirstName = row[customerFirstNameIndex].ToString();
customer.LastName = row[customerLastNameIndex].ToString();
}//end foreach
function AddTHEAD(tableName)
{
var table = document.getElementById(tableName);
if(table != null)
{
var head = document.createElement("THEAD");
head.style.display = "table-header-group";
head.appendChild(table.rows[0]);
table.insertBefore(head, table.childNodes[0]);
}
}
Update:Master.PropertyOrMember="RightHand";